Set cookies in Playwright to bypass cookiepopups
Cookie consent popups can be a pain when scraping websites or running scripts.
If you set cookies in the Playwright script, the consent is automatically accepted, and the popup doesn’t popup.
How to get the cookies that accept the cookieconsent?
- Go to site you work with
- Open Inspector
- Go to Application
- Go to Data
- Clear all data
- Accept the cookieconsent popup
- See which new cookies get set in the inspector
- Export cookies with Edit this Cookie
Edit this cookie Chrome plugin:
https://chrome.google.com/webstore/detail/editthiscookie/fngmhnnpilhplaeedifhccceomclgfbg?hl=nl
(Edit this Cookie is added in the ‘Inspector’ and creates a shortcut in the browser context menu, right click on a page)
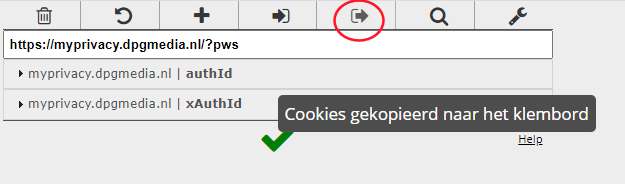
You get code like this:
{
"domain": "myprivacy.dpgmedia.nl",
"expirationDate": 1695326976.860644,
"hostOnly": true,
"httpOnly": false,
"name": "authId",
"path": "/",
"sameSite": "None",
"secure": true,
"session": false,
"storeId": "0",
"value": "8570cd70-a2c8-4c69-a3a6-b38609bdaf57",
"id": 1
},
Paste the copied block in the cookiesArray (paste at top of the script)
const cookiesArr = [{
"domain": "myprivacy.dpgmedia.nl",
"expirationDate": 1695326976.860644,
"hostOnly": true,
"httpOnly": false,
"name": "authId",
"path": "/",
"sameSite": "lax",
"secure": true,
"session": false,
"storeId": "0",
"value": "8570cd70-a2c8-4c69-a3a6-b38609bdaf57",
"id": 1
}
]
and add the ‘await context addCookies’ between context and page, like so:
const context = await browser.newContext({});
await context.addCookies([...cookiesArr])
const page = await context.newPage();
Errors after adding cookies
If you get errors like:
UnhandledPromiseRejectionWarning: browserContext.addCookies: cookies[0].sameSite: expected one of (Strict|Lax|None)
Just change ‘sameSite’ to ‘None’.
Alternative way to get cookies in Playwright
The cookies used on the page should be logged to the console.
const all_cookies = await context.cookies()
console.log('The cookies of the page:', all_cookies)